Vous devez être membre et vous identifier pour publier un article.
Les visiteurs peuvent toutefois commenter chaque article par une réponse.
Java : répertoire de l’application
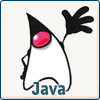
Si dans une application Java nous désirons connaître le répertoire depuis lequel une classe est exécutée, la majorité des sites et forums dans lesquels la question est posée répondent qu’il faut utiliser la propriété système "user.dir".
Ce n’est pas totalement faux, mais cette propriété retourne en réalité le répertoire depuis lequel la commande est lancée.
Exemples
steph@astate:~$cd /media/DATA/steph/test
steph@astate:/media/DATA/steph/test$java -jar myTest.jar
path = /media/DATA/steph/test
jar found : ok
steph@astate:/media/DATA/steph/test$
Dans ce cas, nous positionnons le répertoire actif sur "/media/DATA/steph/test" qui est le répertoire qui contient le jar, puis nous exécutons le jar (qui effectue simplement une sortie de System.getProperty("user.dir"), puis vérifie si le jar se trouve dans le répertoire).
Le résultat affiché correspond bien au répertoire qui contient le jar.
steph@astate:~$java -jar /media/DATA/steph/test/myTest.jar
path = /home/steph
jar found : no
steph@astate:~$
Dans ce cas, nous invoquons la commande sans changer de répertoire actif, et le test échoue, car la propriété "user.dir" correspond au répertoire dans lequel nous nous trouvons au moment de lancer la commande.
Une solution...
Afin d’éviter ce genre de problème, j’utilise une classe statique dans laquelle j’ai placé différents codes nécessaires à la manipulation des fichiers :
Code Java (122 lignes)
/** * {@link java.lang.System#getProperty(String) * System.getProperty("user.dir")} does not return class directory each * time. If we start a java application from a command prompt by giving the * path (not using ‹code›cd‹/code› command to set the active directory), * ‹code›user.dir‹/code› contains the active directory and not the * application directory. * * @param startingClass * class witch we need to know directory * @param cached * ‹ul› * ‹li›‹code›true‹/code› to avoid doing the parsing each time. * The result is stored as cache after the first call.‹/li› * ‹li›‹code›false‹/code› to ignore cached result (this does not * affect the cached result). * * @return path of the class arg * * @since 0.1.12-SNAPSHOT (Feb 12 2009) */ boolean cached) { if (!cached || applicationPath == null) { String classDirectory = "/" + startingClass.getName().replace(’.’, ’/’) + ".class"; // classDirectory = /be/gaudry/model/file/FileHelper.class try { // This class is run from a jar file if (classDirectory.startsWith("jar:file:")) { // classDirectory = // jar:file:/media/DATA/steph/dev/java/projets/dist/EducaBrol-Full-jar-with-dependencies.jar!/be/gaudry/model/file/FileHelper.class // remove "jar:file:" string and classpath string from URL int index = classDirectory.indexOf("!"); classDirectory = classDirectory.substring(9, index); // classDirectory = // /media/DATA/steph/dev/java/projets/dist/EducaBrol-Full-jar-with-dependencies.jar // remove jar name from URL index = classDirectory.lastIndexOf("/"); classDirectory = classDirectory.substring(0, index); // classDirectory = /media/DATA/steph/dev/java/projets/dist } else { // This class is not in a jar file // classDirectory = // file:/media/DATA/steph/dev/java/projets/broldev/core/broldev.core.model/target/classes/be/gaudry/model/file/FileHelper.class // remove file name and extension from URL int index = classDirectory.lastIndexOf("/"); classDirectory = classDirectory.substring(0, index); // classDirectory = // file:/media/DATA/steph/dev/java/projets/broldev/core/broldev.core.model/target/classes/be/gaudry/model/file // remove file: prefix from URL classDirectory = classDirectory.substring(5, classDirectory .length()); // classDirectory = // /media/DATA/steph/dev/java/projets/broldev/core/broldev.core.model/target/classes/be/gaudry/model/file if (null != pack) { // packPath = package be/gaudry/model/file // remove package from URL if (classDirectory.endsWith(packPath.substring(8))) { classDirectory = classDirectory.substring(0, (classDirectory.length() - packPath .length())); // classDirectory = // /media/DATA/steph/dev/java/projets/broldev/core/broldev.core.model/target/ } // remove maven structure from URL if (classDirectory.endsWith(maven)) { classDirectory = classDirectory.substring(0, (classDirectory.length() - maven.length())); // classDirectory = // /media/DATA/steph/dev/java/projets/broldev/core/broldev.core.model/ } } } // Windows path may not start with "/" before drive letter classDirectory = classDirectory.substring(1); } e.printStackTrace(); } if (!cached) { return classDirectory; } applicationPath = classDirectory; } return applicationPath; }

Source : indéterminée
Version en cache
21/07/2025 01:23:34 Cette version de la page est en cache (à la date du 21/07/2025 01:23:34) afin d'accélérer le traitement. Vous pouvez activer le mode utilisateur dans le menu en haut pour afficher la version plus récente de la page.Document créé le 13/09/2004, dernière modification le 26/10/2018
Source du document imprimé : https://www.gaudry.be/ast-rf-448.html
L'infobrol est un site personnel dont le contenu n'engage que moi. Le texte est mis à disposition sous licence CreativeCommons(BY-NC-SA). Plus d'info sur les conditions d'utilisation et sur l'auteur.